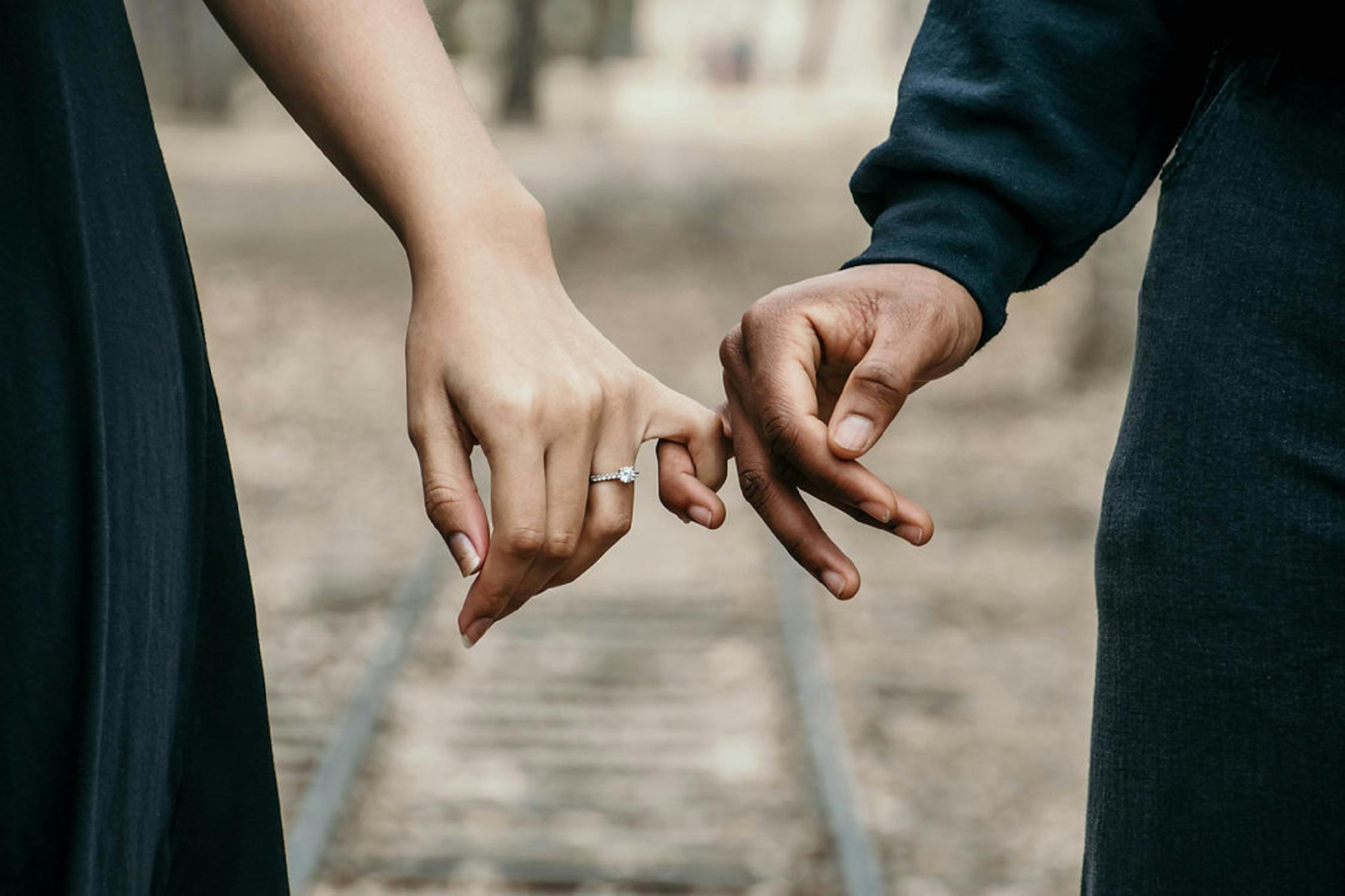
JavaScript Promises
JavaScript Promises
What is a Promise?
In JavaScript, asynchronous operations are a common occurrence. Asynchronous operations may take some time, such as fetching data from a server or reading a file. To handle these asynchronous operations, we use Promises. Promises are objects that represent a value that may not be available yet. This blog post will cover the basics of Promises and provide concise code examples.
A Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. The Promise object has a state which can be fulfilled, rejected, or pending. Once a Promise is fulfilled or rejected, it cannot be changed.
A Promise has two main methods: then()
and catch()
. The then()
method is called when the Promise is fulfilled, and the catch()
method is called when the Promise is rejected.
Creating a Promise
To create a Promise in JavaScript, we use the Promise constructor. The Promise constructor takes a single function as an argument with two parameters: resolve() and reject().
const myPromise = new Promise((resolve, reject) => {
// asynchronous operation
})
The resolve() function is called when the Promise is fulfilled, and the reject() function is called when the Promise is rejected.
Resolving a Promise
To resolve a Promise, we call the resolve() function with a value. The value passed to the resolve() function is the value that will be available to the Promise when it is fulfilled.
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Hello, World!')
}, 2000)
})
myPromise.then((value) => {
console.log(value) // Output: Hello, World!
})
In the above example, we create a Promise that will be resolved after 2 seconds with the value ‘Hello, World!‘. We then use the then() method to log the value to the console.
Rejecting a Promise
To reject a Promise, we call the reject() function with a reason. The reason passed to the reject() function is the reason why the Promise was rejected.
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error('Something went wrong.'))
}, 2000)
})
myPromise.catch((error) => {
console.log(error.message) // Output: Something went wrong.
})
In the above example, we create a Promise that will be rejected after 2 seconds with the reason ‘Something went wrong.’. We then use the catch() method to log the reason to the console.
Chaining Promises
Promises can be chained together using the then() method. The then() method takes two functions as arguments: onFulfilled and onRejected. The onFulfilled function is called if the Promise is fulfilled, and the onRejected function is called if the Promise is rejected.
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Hello, World!')
}, 2000)
})
myPromise
.then((value) => {
console.log(value) // Output: Hello, World!
return value.toUpperCase()
})
.then((value) => {
console.log(value) // Output: HELLO, WORLD!
})
In the above example, we create a Promise that will be resolved after 2 seconds with the value ‘Hello, World!‘. We then use the then() method to chain two functions together. The first function logs the value to the console and returns the value in uppercase. The second function logs the uppercase value to the console.
Handling Errors
To handle errors in a Promise, we use the catch() method. The catch() method takes a function as an argument. The function is called if the Promise is rejected.
const myPromise = new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error('Something went wrong.'))
}, 2000)
})
myPromise.catch((error) => {
console.log(error.message) // Output: Something went wrong.
})
In the above example, we create a Promise that will be rejected after 2 seconds with the reason ‘Something went wrong.’. We then use the catch() method to log the reason to the console.
Conclusion
Promises are a powerful tool in JavaScript for handling asynchronous operations. With the Promise
constructor, we can create Promises, resolve them, reject them, and chain them together. By using the then()
and catch()
methods, we can handle the fulfillment and rejection of Promises. With concise code examples, we have covered the basics of Promises. With practice, we can use Promises to write cleaner, more readable, and more maintainable code.