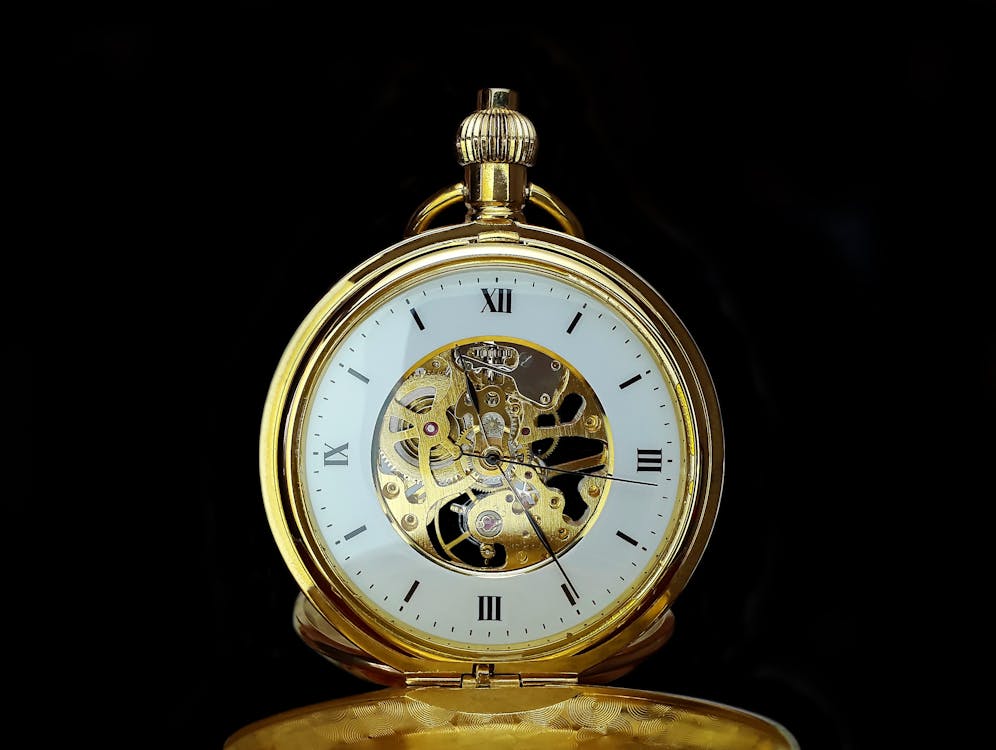
JavaScript Async and Await
JavaScript Async and Await
Introduction
Asynchronous programming is an essential concept in JavaScript that allows for non-blocking code execution. It helps avoid long wait times, affecting the user experience. One way to implement asynchronous programming is by using callbacks, but they can lead to callback hell. This blog post discusses a more modern approach to asynchronous programming in JavaScript: Async and Await.
Async and Await
Async and Await is a new way of writing asynchronous code in JavaScript. It was introduced in ES8 (2017), providing a cleaner and more concise way of handling asynchronous operations. Async and Await are built on top of Promises, a way to handle asynchronous operations in JavaScript.
Async Functions
Async functions are a new function in JavaScript that allows us to write asynchronous code that looks synchronous. When a function is declared as async, it automatically returns a Promise. The syntax for declaring an async function is as follows:
async function functionName() {
// asynchronous code here
}
Await Operator
The await operator is used to wait for a Promise to resolve. It can only be used inside an async function. The syntax for using the await operator is as follows:
async function functionName() {
const result = await promise
}
Code Examples
Let’s look at some code examples to see how Async and Await works in practice.
Example 1: Fetching Data from an API
async function fetchData() {
try {
const response = await fetch('<https://jsonplaceholder.typicode.com/todos/1>')
const data = await response.json()
console.log(data)
} catch (error) {
console.log(error)
}
}
fetchData()
In this example, we’re fetching data from an API using the Fetch API. We’re using Async and Await to handle the asynchronous nature of the fetch request. We’re wrapping the fetch request in a try-catch block to handle any errors that might occur.
Example 2: Using Async and Await with Promises
async function getData() {
const promise1 = Promise.resolve('Hello')
const promise2 = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('World')
}, 2000)
})
const result1 = await promise1
const result2 = await promise2
console.log(result1 + ' ' + result2)
}
getData()
In this example, we’re using Async and Await with Promises. We’re creating two Promises: one using Promise.resolve and another using the Promise constructor. We’re using the await operator to wait for each Promise to resolve before logging the results to the console.
Example 3: Handling Errors with Async and Await
async function fetchData() {
try {
const response = await fetch('<https://jsonplaceholder.typicode.com/todos/1>')
const data = await response.json()
if (!data.title) {
throw new Error('Data not found')
}
console.log(data.title)
} catch (error) {
console.log(error)
}
}
fetchData()
In this example, we’re handling errors with Async and Await. We’re fetching data from an API, and if the data doesn’t have a title property, we’re throwing an error. We’re using the try-catch block to handle the error and log it to the console.
Conclusion
If you’re looking for a better way to handle asynchronous operations in your JavaScript code, then Async and Await might be just what you need. Not only does it provide a cleaner and more concise way of writing asynchronous code, but it also makes it easier to read and maintain. By automatically returning Promises and using the await operator to wait for them to resolve, Async functions help streamline the process of handling asynchronous tasks. So if you want to improve the efficiency and readability of your JavaScript code, give Async and Await a try!