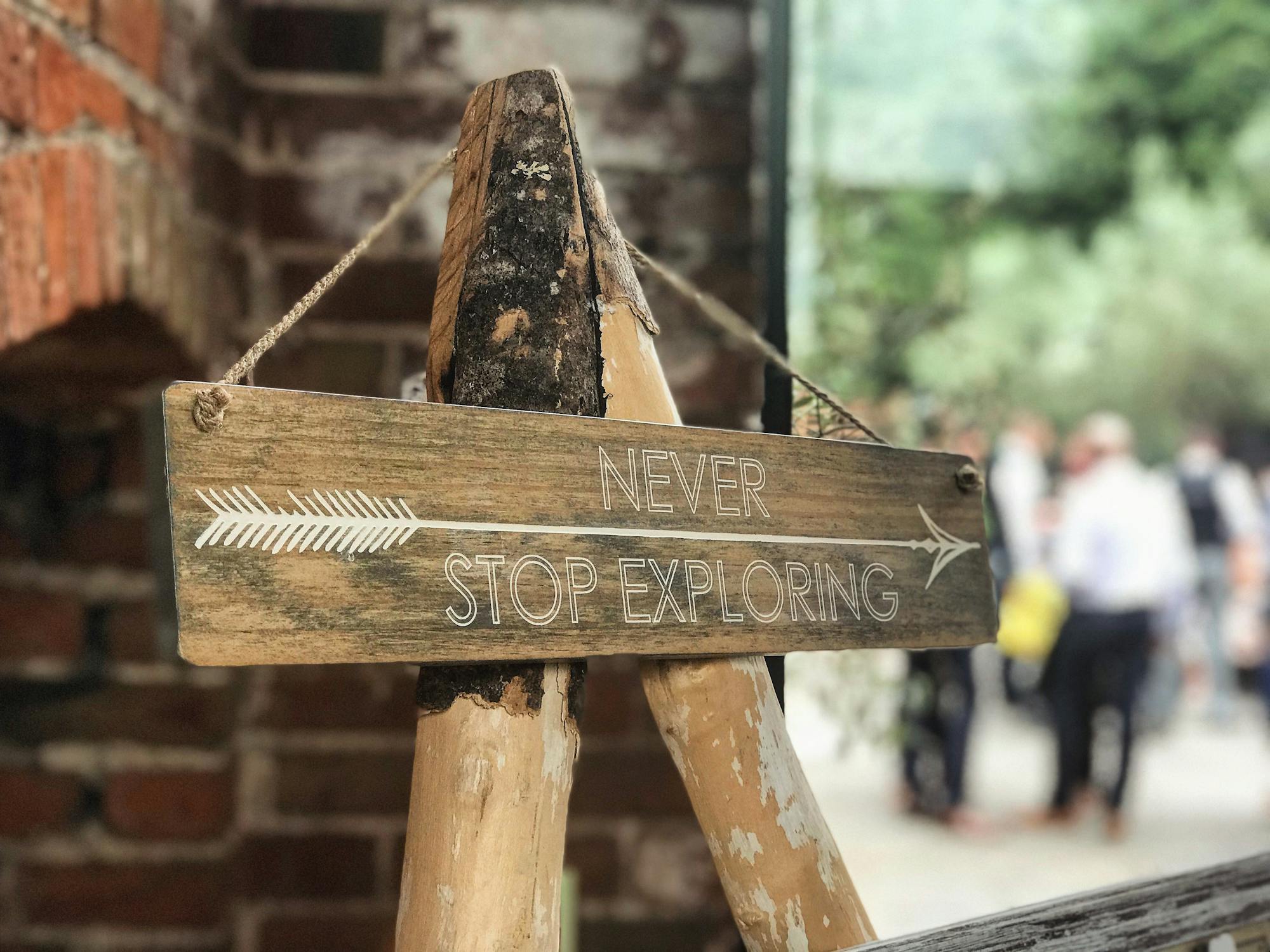
JavaScript Arrow Function
JavaScript Arrow Function
What is an Arrow Function?
JavaScript has introduced arrow functions as a shorthand for writing function expressions. Arrow functions are a more concise way of writing, making code easier to read. This blog post will discuss arrow functions and provide some brief code examples.
In modern JavaScript development, arrow functions have become an essential tool. They offer a concise and powerful way to define functions and make code easier to read and write. Arrow functions are handy when working with array methods and callbacks.
Arrow Function Syntax
Arrow functions are denoted by the “fat arrow” symbol =>. They have a shorter syntax compared to traditional function expressions. Here is an example of a conventional function expression:
let multiply = function (a, b) {
return a * b
}
The same function can be written as an arrow function:
let multiply = (a, b) => {
return a * b
}
The arrow function takes the parameters a
and b
and returns their product. The curly braces and return
keyword are not needed when there is only one expression in the function body.
Arrow functions can also be used for more complex functions. Here is an example of an arrow function that calculates the sum of an array of numbers:
let numbers = [1, 2, 3, 4, 5]
let sum = numbers.reduce((acc, curr) => acc + curr)
console.log(sum) // 15
The reduce method takes an array and applies a function to each element, returning a single value. In this example, the arrow function takes two parameters: acc (the accumulated value) and curr (the current value) and returns their sum.
Arrow Functions with Array Methods
Arrow functions can be used with array methods such as map, filter, and reduce. Here is an example of using the map method with an arrow function:
let numbers = [1, 2, 3, 4, 5]
let squaredNumbers = numbers.map((num) => num * num)
console.log(squaredNumbers) // [1, 4, 9, 16, 25]
The map
method takes an array and applies a function to each element, returning a new array with the modified elements. In this example, the arrow function takes a number and returns its square.
Arrow functions can also be used with the filter
method to filter elements based on a condition. Here is an example that filters out even numbers from an array:
let numbers = [1, 2, 3, 4, 5]
let oddNumbers = numbers.filter((num) => num % 2 !== 0)
console.log(oddNumbers) // [1, 3, 5]
The arrow function takes a number and returns true if it is odd and false if it is even. The filter method returns a new array with the elements that passed the condition.
Arrow Functions with Object Methods
Arrow functions can also be used with object methods. Here is an example of defining an object method as an arrow function:
let person = {
name: 'John',
age: 30,
greet: () => {
console.log(`Hello, my name is ${this.name}`)
}
}
person.greet() // Hello, my name is undefined
The arrow function takes no parameters and returns undefined. When the greet method is called, it logs the string “Hello, my name is undefined” to the console. This is because the arrow function does not have access to the object’s properties.
Here is an example of defining an object method as a regular function:
let person = {
name: 'John',
age: 30,
greet: function () {
console.log(`Hello, my name is ${this.name}`)
}
}
person.greet() // Hello, my name is John
Conclusion
In conclusion, arrow functions are a concise and powerful feature of JavaScript. They make code easier to read and write, especially when used with array methods. Arrow functions offer a more streamlined syntax than traditional function expressions and are especially useful for lambda-style functions that don’t require a lot of logic.
It’s important to remember that arrow functions do not have their own this
value, so they should not be used as object methods. When working with object methods, it’s best to use traditional function expressions to ensure that this
refers to the correct object.
Overall, arrow functions are an essential tool for modern JavaScript development. They offer a concise, easy-to-read syntax and are useful for a wide range of use cases. If you’re not already using arrow functions in your JavaScript code, it’s definitely worth giving them a try!