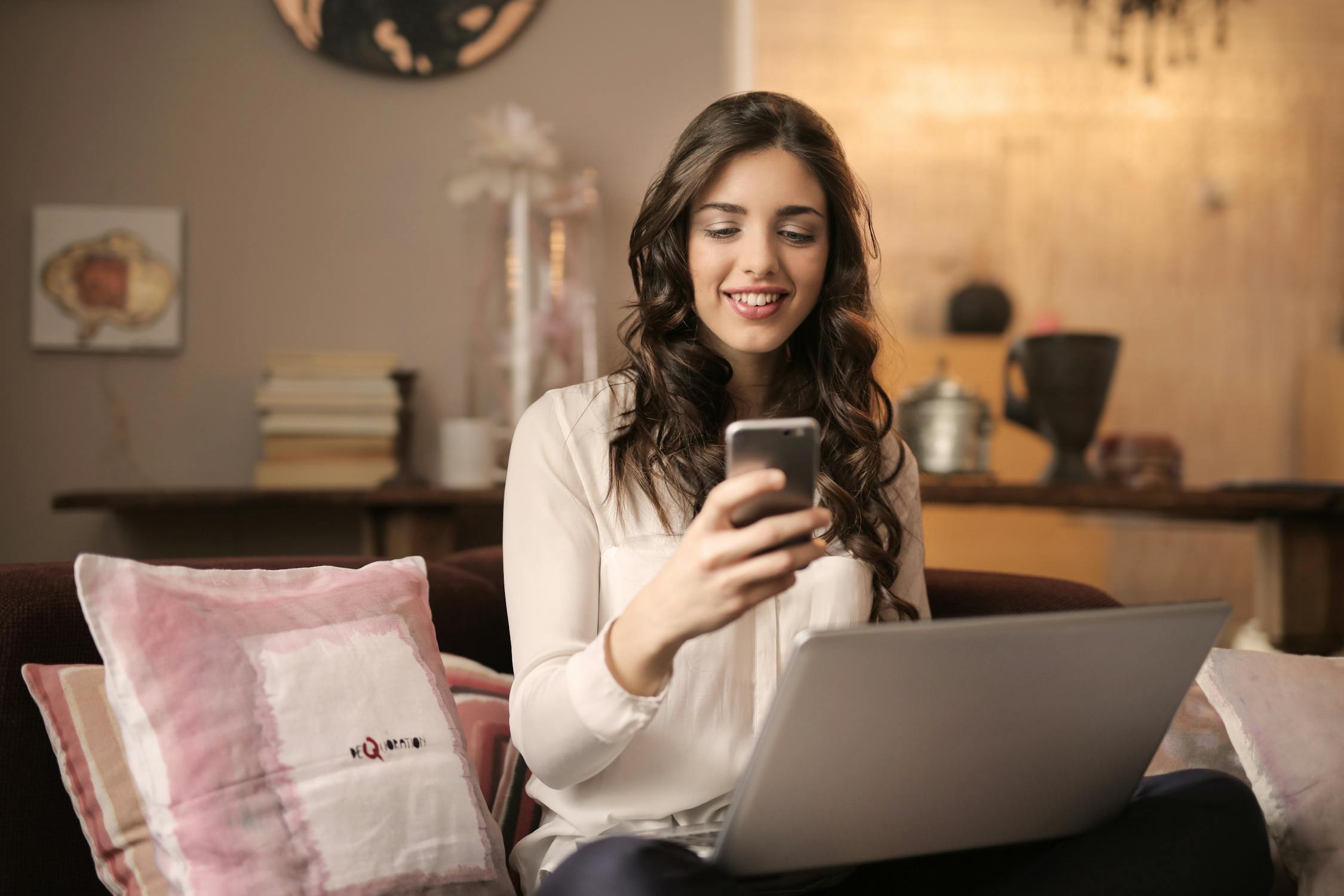
Understanding JavaScript Callbacks
Understanding JavaScript Callbacks
What are JavaScript Callbacks?
A callback is a function passed as an argument to another function. The function that receives the callback executes it at some point during its execution. Callbacks are commonly used in JavaScript programming to handle asynchronous operations, such as making an HTTP request or reading a file from a disk.
Callbacks are essential to JavaScript programming, as they allow us to write code that can handle events and execute tasks asynchronously. Without callbacks, our programs would be limited to running one task at a time, making our code slower and less efficient.
How do JavaScript Callbacks Work?
When a function receives a callback as an argument, it stores the callback in a variable. The function executes its code, and when it is ready to execute the callback, it calls the function that was passed in. The callback function can take arguments passed from the calling function.
Here’s an example of how callbacks work:
function doSomething(callback) {
// execute some code
callback(arg1, arg2)
}
function callback(arg1, arg2) {
// execute some code
}
doSomething(callback)
In the above example, the doSomething
function takes a callback as an argument. When it is ready to execute the callback, it calls the callback
function and passes in arg1
and arg2
. The callback
function can then execute its own code using the values of arg1
and arg2
.
Here’s a better example of how callbacks work:
function doSomething(callback) {
setTimeout(() => {
callback('Hello World!')
}, 1000)
}
function callback(message) {
console.log(message)
}
doSomething(callback)
Or:
function fetchData(url, callback) {
fetch(url)
.then((response) => response.json())
.then((data) => callback(data))
.catch((error) => console.error(error))
}
function handleData(data) {
return data
}
fetchData('<https://api.example.com/data>', handleData)
In the above example, the fetchData function takes a URL and a callback as arguments. It uses the fetch API to make an HTTP request to the given URL and parse the response as JSON. When the data is ready, the callback function is called, and the parsed data is passed in as an argument. The handleData function can then use this data to perform some action.
Why are JavaScript Callbacks Important?
JavaScript is a single-threaded language, which means it can only execute one task at a time. This can cause problems when working with asynchronous operations, which can take a long time to complete. Callbacks allow us to handle these operations without blocking the main thread of execution. This makes our code more efficient and responsive.
In addition, callbacks make it easy to write code that can handle events and execute tasks asynchronously. This is especially important in web development, where we often need to wait for user input or data from external sources before executing certain tasks.
Conclusion
JavaScript callbacks are a powerful feature that allow us to handle asynchronous operations in an efficient and responsive way. By passing functions as arguments, we can execute code at specific points in our program’s execution. This makes our code more flexible and easier to maintain.
Callbacks are an essential part of JavaScript programming, and mastering their use is key to writing efficient and responsive web applications. With the knowledge and understanding of how callbacks work, you can take full advantage of JavaScript’s capabilities and create amazing applications that can handle anything that comes their way.